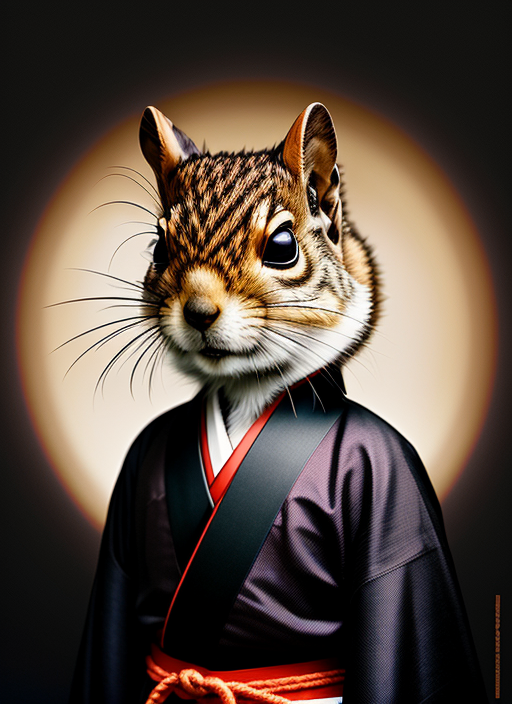
Fibonacci Series in Java
Printing a Fibonacci series in Java is one of the most basic java interview questions, usually, asked in entry-level interviews. In this tutorial, we will study some effective ways to approach this problem in Java.
If you are new to this concept, let me first explain what the Fibonacci series is, before proceeding to the algorithms and the code.
In the case of a Fibonacci sequence, the next number is the sum of the two previous ones. Such as 0, 1, 1, 2, 3, 5, 8, 13, 21, etc. The first two Fibonacci Sequence numbers are 0 and 1.
First term = 0
Second term = 1
Third term = First + Second = 0+1 =1
Fourth term = Second + Third =1+1 = 2
Fifth term = Third + Fourth = 2+1 = 3
Sixth term = Fourth + Fifth = 3+2 = 5 and so on….
There are multiple ways to achieve the output. Let us discuss each one of them one by one.
Content
Fibonacci Series in Java using Loops
In Java, iteration is a process used to continuously go through a code block until a given condition is met. The iterative method is one of the most common methods to solve such problems. Here we are using the ‘for’ loop, you can use the ‘while’ or ‘do-while’ loop, as per your convenience.
package com.artoftesting.java;
public class FibonacciSeries {
public static void main(String args[]) {
//Declare and initialize the variables
int first=0, second=1, third;
//Number of terms in Fibonacci series
int n=10;
//Printing first and second numbers
System.out.print(first + " " + second);
//Loop starts from 2 because 0 and 1 are already printed
for(int i=2; i
Output – 0 1 1 2 3 5 8 13 21 34
Fibonacci Series in Java using Recursion
The dictionary meaning of recursion is “the repeated application of a recursive procedure or definition.” In Java, if a function calls itself multiple times as a subroutine(methods), such an approach is called a recursive method.
It is important to know a recursive approach to a problem as it breaks down a code into small repetitive blocks. Thus making it easier to understand by making the code look less complicated.
package com.artoftesting.java;
public class FibonacciSeries {
static int first=0, second=1, third=0;
static void fibonacci(int n){
if(n>0){
third = first + second;
first = second;
second = third;
System.out.print(" " + third);
//Calling the method recursively
fibonacci(n-1);
}
}
public static void main(String args[]){
//Number of terms in Fibonacci series
int n=10;
//Printing 0 and 1
System.out.print(first + " " + second);
//n-2 because 2 numbers are already printed
fibonacci(n-2);
}
}
Output – 0 1 1 2 3 5 8 13 21 34