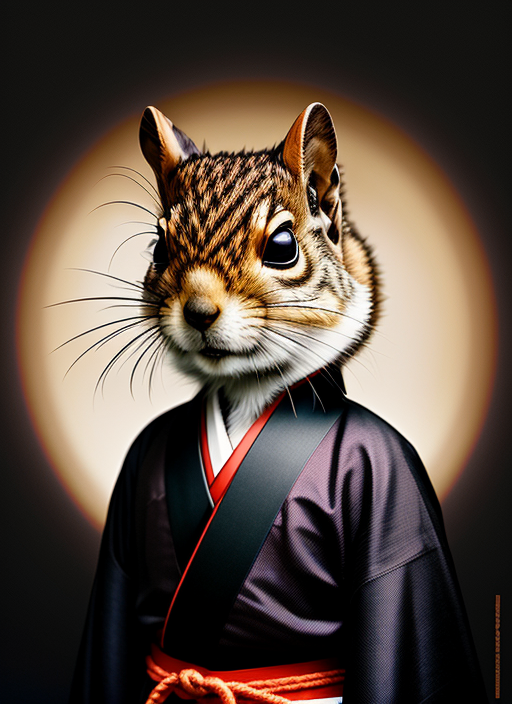
Locators in Selenium WebDriver
Hello friends! continuing with our “Selenium Automation” series, in this post we will study the different types of locators available in Selenium. Before studying the different locators, let’s first see the need for locators in the automation process.
A simple automation process in Selenium can be presented as-
- Launching browser
- Opening the desired website to be automated
- Locating web elements like a textbox
- Performing operations on the located web elements like writing in the textbox
- Performing assertion like checking ‘Success’ message
Now, let’s see a sample code snippet implementing the above process. We will be studying each line of code in the coming tutorials. For now, just consider it as a black-box and focus on the operations performed(specified in the comments).
//Launching Firefox browser
WebDriver driver = new FirefoxDriver();
//Opening google.com
driver.get("http://www.google.com");
//Initializing webelement searchBox
WebElement searchBox = driver.findElement(By.name("q"));
//Writing a text "ArtOfTesting" in the search box
searchBox.sendKeys("ArtOfTesting");
So, here we see that in order to perform an operation on web element – searchBox, we first need to locate it. Here, By.name(“q”) is a locator which when passed to findElement method returns a searchBox web element.
Before going further with the different types of locators available in Selenium, let’s first see, how to get the different attributes of an element that are used in the locators.
Content
Using Developer Tool
Locating web elements requires knowledge of their HTML attributes. For the HTML source code of specific elements, we can use the inbuilt developer tool (launched by pressing f12 in a browser).
Steps for finding element’s HTML attributes-
- Launch the website to be automated e.g. – https://www.google.com
- Press F12 to launch the developer tool.
- Click on the inspect-element icon as displayed in the image below.
- After clicking on the inspect-element icon, click on the web element to be located e.g. Google Search box. Once we click on the element, its HTML will get displayed in the firebug UI.
- Here, we can see the different attributes of the web elements like – id, class, name, along with its tag like input, div, etc. Now, we will be using these tags, attributes, and values to locate elements using different locators.
Locators in Selenium
There are a total of 8 locators in Selenium WebDriver-
1. By Id – Locates element using id attribute of the web element.
WebElement element = driver.findElement(By.id("elementId"));
2. By className – Locates the web element using className attribute.
WebElement element = driver.findElement(By.className("objectClass"));
3. By tagName – Locates the web element using its HTML tag like div, a, input etc.
WebElement element = driver.findElement(By.tagName("a"));
4. By name – Locates the web element using name attribute.
WebElement element = driver.findElement(By.name("male"));
5. By linkText – Locates the web element of link type using their text.
WebElement element = driver.findElement(By.linkText("Click Here"));
6. By partialLinkText – Locates the web element of link type with partial matching of text.
WebElement element = driver.findElement(By.partialLinkText("Click"));
7. By cssSelector – Locates the web element using css its CSS Selector patterns(explained in detailed here – CSS Locators).
WebElement element = driver.findElement(By.cssSelector("div#id"));
8. By xpath – Locates the web element using its XPaths(explained in detailed here XPath Locators).
WebElement element = driver.findElement(By.xpath("//div[@id='id']"));
Now you have successfully learned how to locate elements in Selenium. As you can see locating element by id, className, tagName, name, linkText, and partialLinkText is simple. We just have to select the right locator based on the uniqueness of the element e.g. we prefer using id because the id of elements is generally unique. But there can be scenarios where we might not have id attributes of web elements, also other locators like name, className might not fetch the unique required web element. In those scenarios, we should use CSS selector and XPath locators.
These locators are very powerful and help in creating robust locators for complex web elements. We have dedicated separate tutorials for these two locators in our coming posts- CSS Locators and XPath Locators. You are advised to have a basic understanding of both the locators and choose either of the locators for detailed study.