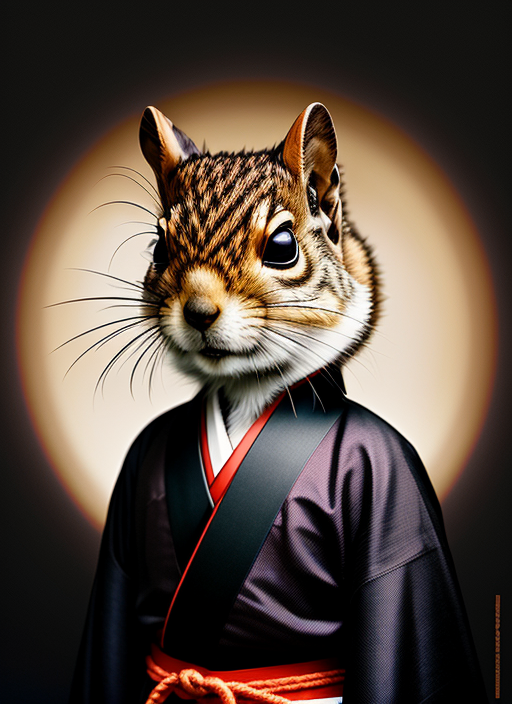
Connect to MongoDB in Java
MongoDB is an open-source, NoSQL database that stores data in the form of documents. Being a NoSQL database, it is not relational and does not comply with ACID properties – Atomicity, Consistency, Isolation, and Durability.
Content
MongoDB Driver
For connecting to MongoDB, we need a MongoDB java driver. The Java driver can be downloaded from here. It is required to be added to the classpath or as a dependency in your maven project’s POM file.
MongoDB Automation with Java
First, we will connect to the MongoClient using the below line of code.
MongoClient mongoClient = new MongoClient( "localhost" , 27017);
Then we will select the DB and collection, we want to query on.
DB db = mongoClient.getDB("dbName");
DBCollection dbCollection = db.getCollection("collectionName");
After that, we will create a search query and fire it to get the DBCursor object.
BasicDBObject searchQuery = new BasicDBObject();
searchQuery.put("key", "value");
DBCursor cursor = dbCollection.find(searchQuery);
Now, we can get the response from the DBCursor object and use the desired assertions to carry out the database testing.
Code snippet
In the following sample code, we will connect to a mongoDB database, execute a find query and then assert the fetched result with expected value.
package com.artOfTesting.mongo;
import com.mongodb.MongoClient;
import com.mongodb.DB;
import com.mongodb.DBCollection;
import com.mongodb.BasicDBObject;
import com.mongodb.DBCursor;
import org.testng.Assert;
public class MongoAutomation{
public static void main( String args[] ){
try{
//Connecting to the mongoDB instance
MongoClient mongoClient = new MongoClient( "localhost" , 27017 );
//Selecting the database
DB db = mongoClient.getDB("dbName");
//Selecting the collection
DBCollection dbCollection = db.getCollection("collectionName");
//Setting search query with the required key-value pair
BasicDBObject searchQuery = new BasicDBObject();
searchQuery.put("key", "value");
//DBCursor with the find query result
DBCursor cursor = dbCollection.find(searchQuery);
//Fetching the response
String response = null;
try {
while(cursor.hasNext()) {
response = response.concat(cursor.next().toString());
}
}
finally {
cursor.close();
}
//Asserting the fetched response with expected value
Assert.assertTrue(response.contains("ExpectedValue"));
}
catch(Exception e){
System.out.println(e.getMessage());
}
}
}