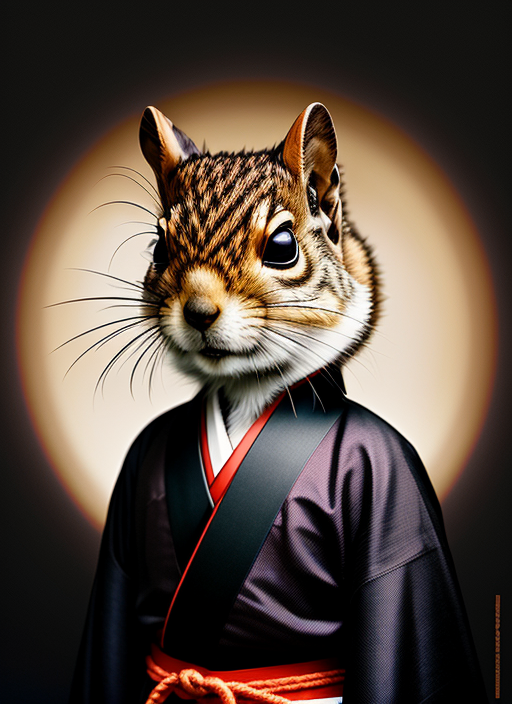
Rest API Automation in Java – Get Method
Content
Using Rest-assured and TestNG
In this post, we will learn to automate REST APIs using the Rest-Assured library and TestNG. Rest Assured is a Java library using which we can test and validate the REST web services. Although Rest-assured provides its own validating mechanism(assertions for validating response) but we can combine Rest-assured with TestNG to get the best of both the libraries.
During the course of this tutorial, we will be using the following-
- Sample Rest API to be used in Automation – RestCountries API
- Rest-Assured and its dependencies (https://code.google.com/p/rest-assured/wiki/Downloads) (Remember to download and add to the class path, the other dependencies also). POM dependencies for the Rest Assured and its dependencies-
com.jayway.restassured
rest-assured
2.3.4
org.codehaus.groovy
groovy-all
2.3.5
- TestNG as a testing framework
Rest API Get Method Automation in Java
In the following example, we are using the Rest-assured library’s “get” method to make an HTTP Get request to the restcountries.eu API- http://restcountries.eu/rest/v1/name/{countryName} that fetches the capital of a country. Then we will fetch the response in JSONArray and use TestNG’s assert method for validation.
You can also download the RestAPI Automation sample script from API Automation Script.
package RestAPITest;
import static com.jayway.restassured.RestAssured.get;
import org.json.JSONArray;
import org.json.JSONException;
import org.testng.Assert;
import org.testng.annotations.Test;
import com.jayway.restassured.response.Response;
public class RestTest {
@Test
public void getRequestFindCapital() throws JSONException {
//make get request to fetch capital of norway
Response resp = get("http://restcountries.eu/rest/v1/name/norway");
//Fetching response in JSON
JSONArray jsonResponse = new JSONArray(resp.asString());
//Fetching value of capital parameter
String capital = jsonResponse.getJSONObject(0).getString("capital");
//Asserting that capital of Norway is Oslo
Assert.assertEquals(capital, "Oslo");
}
}
In our next tutorial – Rest API POST method automation in Java, we will study the HTTP post method automation using the Rest-assured library in Java.