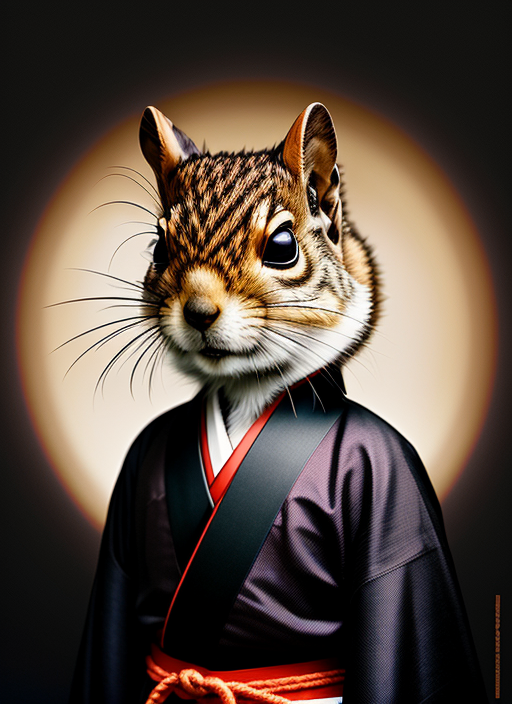
Handling Cookies in Selenium WebDriver
This post is dedicated to the handling of cookies using Selenium webDriver. We will be studying cookies, the need of automating cookie handling scenarios and different methods provided by Selenium to add, get and delete cookies.
Content
What are Cookies?
Cookies are files stored in local computers containing information submitted by the websites visited by a user. The information is stored in key-value pairs and allows a particular website to customize its content as per the user.

Need for Handling Cookies in Automation
During automation, we might need to handle cookies for the following reasons-
- There could be scenarios specific to cookies that need to be automated and requires validating entries in cookie files.
- If we could create and load cookie files during automation, we can skip some scenarios like login to the application for each test case as the same gets handled by cookies. E.g. in some applications like GMail, we only need to login once, after that the login information gets stored in cookies and loaded each time we open the Gmail link. This saves the overall test execution time.
Get Cookies in Selenium
We can get Cookies in Selenium WebDriver in two ways-
- getCookieNamed(String cookieName) – To get a particular Cookie by name
- getCookies() – To get all the Cookies in the current domain.
//To fetch a Cookie by name
System.out.println(driver.manage().getCookieNamed(cookieName).getValue());
//To return all the cookies of the current domain
Set cookiesForCurrentURL = driver.manage().getCookies();
for (Cookie cookie : cookiesForCurrentURL) {
System.out.println(" Cookie Name - " + cookie.getName()
+ " Cookie Value - " + cookie.getValue()));
}
Add Cookies in Selenium
addCookie(Cookie cookie) – To add a particular Cookie in Selenium WebDriver.
Cookie cookie = new Cookie("cookieName", "cookieValue");
driver.manage().addCookie(cookie);
Delete Cookies in Selenium
Delete Cookies in Selenium WebDriver can be done in three ways-
- deleteCookieNamed(String cookieName) – To delete a particular Cookie by name.
- deleteCookie(Cookie cookie) – To delete a particular Cookie.
- deleteAllCookies() – To delete all the Cookies.
//Delete a particular Cookie by name
driver.manage().deleteCookieNamed(cookieName);
//Delete a particular Cookie
driver.manage().deleteCookie(cookie);
//Delete all the Cookies
driver.manage().deleteAllCookies();
That’s all we have in this section. Please comment below to share your views or ask any questions and don’t forget to check our complete step-by-step selenium tutorial here.